SLTwitCtrl ActiveX Control for Twitter - Example Code
Example 1 - Visual Basic -
Preparing the Twitter control for communication
Before
you can send a tweet to Twitter you must specify some information that
Twitter requires for communication. You must specify an application
name, a consumer key, a consumer secret, a username and a password. You
can do this by setting corresponding properties in the ActiveX control.
You can do it via the Properties window in
Microsoft Visual Basic (as shown in the
picture to the right) or by specifying the information programmatically,
as shown in the Visual Basic example below:
|
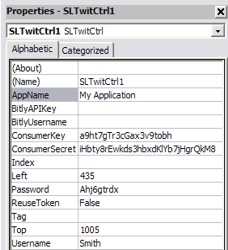 |
' Specify application name
SLTwitCtrl1.AppName
=
"My Application"
' Specify consumer key and consumer secret
SLTwitCtrl1.ConsumerKey
=
"a9ht7gTr3cGax3v9tobh"
SLTwitCtrl1.ConsumerSecret
=
"iHbty8rEwkds3hbxdKlYb7jHgrQkM8"
' Specify username and password
SLTwitCtrl1.Username
=
"Smith"
SLTwitCtrl1.Password
=
"Ahj6gtrdx"
|
|
A consumer key and consumer secret can be retrieved from Twitter's
website for developers. On the same website you can register your
application (which is necessary before you can send tweets to Twitter).
Open this web page
to read more.
Example 2 - Visual Basic - Send a message (a
tweet) to Twitter
This example in Visual Basic shows how to send a
message (a tweet) to Twitter. It also shows how to handle errors, if
something goes wrong.
Dim bStatus As Boolean
Dim nErrorCode As Integer
Dim sReplyMsg As String
Dim sErrMsg As String
' Post a tweet
bStatus = SLTwitCtrl1.TwitPostMessage("This is a tweet!")
' Check if an error occurred
If bStatus = False Then
' Get error code
nErrorCode = SLTwitCtrl1.TwitGetLastError()
sErrMsg = "Error #" + CStr(nErrorCode) + " occurred!"
If nErrorCode > 1000 Then
' Get response from Twitter
SLTwitCtrl1.TwitGetLastResponse
sReplyMsg
sErrMsg = sErrMsg + " Twitter
returned: " + sReplyMsg
Else
MsgBox sErrMsg, vbExclamation,
"Error"
End If
Else
MsgBox "The message was successfully sent to Twitter!",
vbInformation, "Information"
End If |
Example 3 - Visual Basic - How to use
Bitly to
shorten a URL in a message
This example in Visual Basic shows
how to shorten a URL in a message by using the
TwitShortenURLInMessage method in the SLTwitCtrl ActiveX
control. The Bitly service is used.
Dim sMessageOriginal As String
Dim sMessageShortenedURL As String
' Create a message that contains a URL
sMessageOriginal = _
"Read more about MultiMailer here:
http://www.samlogic.net/multimailer/multimailer.htm"
' Now shorten the URL in the message
sMessageShortenedURL =
SLTwitCtrl1.TwitShortenURLInMessage(sMessageOriginal, 1)
' Now display the updated message in a message box
MsgBox sMessageShortenedURL, vbInformation, "Message with
shortened URL" |
The message text "Read more about MultiMailer here:
http://www.samlogic.net/multimailer/multimailer.htm" will be updated
to have the following contents: "You can read more about the Twitter
component here: http://bit.ly/13i0sOE". The image below shows how
the message box that is opened in the last line of the example look
like:
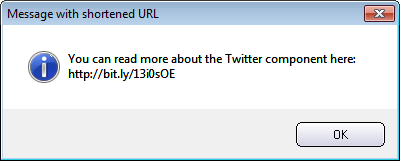
< Go back
|
|